|
The programs that you turn in should conform to the style guide contained in the first of the following three links and they will be graded according to the criteria given in the second link. The third link below gives detailed instructions on exactly how you are to turn in your finished assignments.
Java Language Coding Guidelines
Program grading criteria
Turning in your assignments
The style guide is a PDF file; you should print it out and keep it for reference while you are programming. It was written by Cay Horstmann, a well known Java author. Two other style guides that you might also find useful are avoiding style crime, by Gregory Rawlins, and Code Conventions for the Java Programming Language
, by Sun.
Below are your Java programming assignments. The due date for each one is contained in the assignment description.
- NOTE:
- The exam, which is Thursday at 6:00 p.m., is supposed to be in G011, the room that we meet in on Tuesdays.
-
Practice Exam.
- Here is a practice exam for the exam on Thursday, December 14.
-
Assignment 8.
- This assignment is a slight modification of Programming Exercise 15.2 on page 665. Write a program that will count the number of lines, the number of words, and the number of characters in a file. The filename should be passed as a command-line argument, as shown in Figure 15.21 (if there is no command-line argument, then your program should output an appropriate error message and then quit). Your program should have exception handlers for FileNotFoundException and IOException. This assignment is due Saturday, Decmber 16.
Hint: Do not do this program the hard way, which would be to read the input file one character at a time. Make Java do as much of the work as possible. For example, read the file one line at a time using a readLine() method. Then count how many times you need to call readLine() and that is the number of lines in the file. For each line that you read in, use the length() method from the String class to find out how many characters are in the line. And for each line that you read in, create a StringTokenizer object for it and use the countTokens() method from class StringTokenizer to tell you how many tokens (i.e., words) are in the line. See the example program WriteReadDataGUI.java that we went over in class for an example of a program that reads a file line by line and creates a StringTokenizer for each line.
-
Assignment 7.
- Do Programming Exercise 9.11 on page 422 of the textbook. Notice that the button in the GUI has an icon on it. This is the same icon used in the example from page 359 and it is included with the source files that the book's publisher provides from the textbook's web site. Also, to figure out what the GUI in Exercise 9.11 means by "Position Alignment" and "Text Position Alignment", see page 356. Be sure to implement the GUI as shown in Figure 9.42, including the borders around the sub-panels in the GUI. This is due Friday, December 1.
-
Assignment 6.
- Write a program that creates a GUI that looks exactly like the image below.
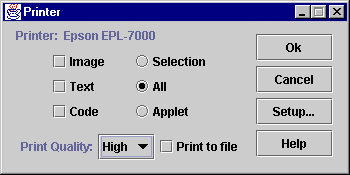
Besides looking like the above image, your program should also put the three radio buttons in a group so that the buttons are mutually exclusive, and the combo box should contain three items, "High", "Medium", and "Low". In addition, implement a listener for each component in the GUI. (You do not have to implement separate listeners for every component; you can have a listener listen for as many components as you like.) The listeners should handle an event by just printing the event object to System.out. This is due Friday, November 17.
-
Assignment 5.
- This assignment is a combination of Programming Exercises 8.1 and 8.4 from pages 347-348 of our textbook.
Create the graphical user interface shown in Figure 8.38 on page 348. This user interface is made up of a frame and two panels with three buttons in each panel. You are to create the panels as described in Programming Exercise 8.4, that is, define a subclass of JPanel that defines a panel with three buttons, and then instantiate two of these panels for your user interface (be sure to get the labels on the buttons numbered correctly). Both your frame and your panels should use the FlowLayout . When a button from the user interface is clicked, a message should be displayed on the console specifying which button was clicked. You should also provide an appropriate implementation of the WindowListener interface and register it with the frame so that the frame will close properly when the Window Close button in the upper-right hand corner of the frame is clicked.
This assignment is due on Friday, November 3.
-
Practice Exam.
- Here is a practice exam for the in-class exam next Tuesday (October 17). This practice exam is a bit longer than what the in-class exam will be.
-
Assignment 4.
- For this assignment you are to write an abstract class
Worker and two concrete subclasses HourlyWorker and SalariedWorker . Each of these classes is described below.
The abstract Worker class has a name field and a salary rate (in dollars per hour) field. The Worker class has an abstract instance method computeWeeklyPay(int hours) . The Worker class should have appropriate constructors, appropriate set and get methods for the name and salary fields, and it should also have a toString method that returns the name and salary rate in an appropriate string.
The HourlyWorker class has a boolean instance field called isUnionMemeber and a String instance field called unionName (which should be set to null if isUnionMemeber is false ). The HourlyWorker class has a computeWeeklyPay(int hours) method that implements the same method from the super class (an hourly worker gets paid for the actual number of hours worked, with overtime over 40 hours paid at time and a half). The HourlyWorker class should have appropriate constructors (that make use of the super class constructors), appropriate set and get methods, and it should also have a toString method that both overrides and calls the toString method in the super class, and that returns the name, salary rate, and union status in an appropriate string.
The SalariedWorker class has an int instance field called subordinates that records the number of workers that the salaried worker manages. The SalariedWorker class has a computeWeeklyPay(int hours) method that implements the same method from the super class (a salaried worker always gets paid for 40 hours, no matter what the actual hours worked is). The SalariedWorker class should have appropriate constructors (that make use of the super class constructors), appropriate set and get methods, and it should also have a toString method that both overrides and calls the toString method in the super class, and that returns the name, salary rate, and number of subordinates in an appropriate string.
Write a test class called TestWorker . It should create an array of type Worker and fill it with some HourlyWorker and some SalariedWorker objects. Then the program should use polymorphism to compute the pay and print out all the information for each object in the array. The test class should also show that your constructors, get, and set methods work.
When you are done you will have four program files, Worker.java , HourlyWorker.java , SalariedWorker.java and TestWorker.java . Put these four files together into one zip file using WinZip. The zip file should have the name CIS263Ass4Surname.zip . Turn in you assignment by attaching this zip file to your e-mail message. (If you need help using WinZip, please ask me after class one day.) This assignment is due on Monday, October 23.
-
Assignment 3.
- Implement a class
Car . A Car object should have two fields, one for fuel efficiency (measured in miles per gallon) and one for fuel level (measured in gallons). The fuel efficiency of a car should be specified as a parameter in the Car constructor and the constructor should set the fuel level to zero. There should be getFuelEfficiency , setFuelEfficiency , getFuelLevel , and setFuelLevel methods. There should also be an addFuel method which adds a specified amount to the fuel level and returns the current fuel level. There should be a drive method which simulates driving the car a specified distance. The drive method should adjust the fuel level by the amount of fuel used and it should return the number of miles driven, which may be less than the number of miles specified if there is not enough fuel.
Write a class CIS263Ass3Surname that tests your Car class. The test class should prompt a user for a fuel efficiency, construct a Car object, prompt the user for an amount of fuel, put the fuel in the Car object, prompt the user for a distance to travel, drive the appropriate distance, and then report back to the user either the fuel level in the car or the distance actually travelled depending on whether or not there was enough fuel in the car to drive the requested distance. (Your test program does not have to do these steps in the exact order given. Organize your test program however you think is best.) Your test program should let the user drive the car until the user enters a distance of zero. Then your test program should prompt the user for an amount of fuel to add to the car and then let the user drive the car some more. If the user enters zero for the amount of fuel to add, then your test program should prompt the user for a new fuel efficiency and construct a new Car object and let the user drive the new car. Your program should terminate if the user enters zero for the fuel efficiency.
Put your two classes into one file with the name CIS263Ass3Surname.java . Put the Car class first. The test class should have a public modifier and the Car class should have no modifier. This assignment is due Friday, October 6.
-
Assignment 2.
- This assignment is a variation on Programming Exercise 4.4 from page 142 of the textbook. Write a method to convert Celsius too Fahrenheit using the following declaration
public static double celsToFahr(double cels)
Write another method using the following declaration
public static void printTable(double celsLow, double celsHigh, int n)
that uses a for-loop and calls the method celsToFahr to print out a table of temperature conversions, like the one shown on page 142, with n number of rows in the table and the Celsius temperatures running from celsLow to celsHigh . So, for example, the call
printTable(0, 10, 6)
should produce the following table.
Cels. Temp Fahr. Temp.
------------------------------------
10.0 50.0
8.0 46.4
6.0 42.8
4.0 39.2
2.0 35.6
0.0 32.0
And the call printTable(1,3,5) should produce the following table.
Cels. Temp Fahr. Temp.
-------------------------------------
3.0 37.4
2.5 36.5
2.0 35.6
1.5 34.7
1.0 33.8
(Do not worry if your columns do not line up correctly. We have not yet learned how to format output.) Write a program that asks a user to enter values for n , celsLow , and celsHigh and then calls printTable . Your program should continue to prompt the user for n , celsLow , and celsHigh and call printTable until the user inputs zero for n . This assignment is due Friday, September 22 (the first day of Autumn).
-
Assignment 1.
- In this assignment you will need to use material from Chapters 2 and 3 of our textbook. Write a Java application that prompts the user to input an integer, reads in the integer
n , and prints out either n plus signs followed by n if the input n is positive, or -n-1 minus signs followed by n if the input n is negative.
So for example, if n is 5, then the output would be
+++++5
(that is, five plus signs followed by 5), and if n is -5, then the output would be
-----5
(that is, five minus signs followed by 5). Your program should continue to read in integers and output one line per integer until the input is the number zero. Your program should terminate when the input is the number zero. Your program should use the methods in the MyInput.java class, from page 58 of the textbook, to do input from the keyboard; this is explained in Chapter 2, pages 58-60. You do not need to do any checking of the input to make sure it is an integer. It is OK (at this point in the course) if your program cannot handle non-integer inputs. This is due Friday, September 8.
|
|